Give your embedded content a serious upgrade! Syncing metadata ensures important details like dates, locations, and descriptions travel along with it.
Ready to learn how?
Let’s modify your AI Engine embedding module – I’ll walk you through it using an event from The Events Calendar:
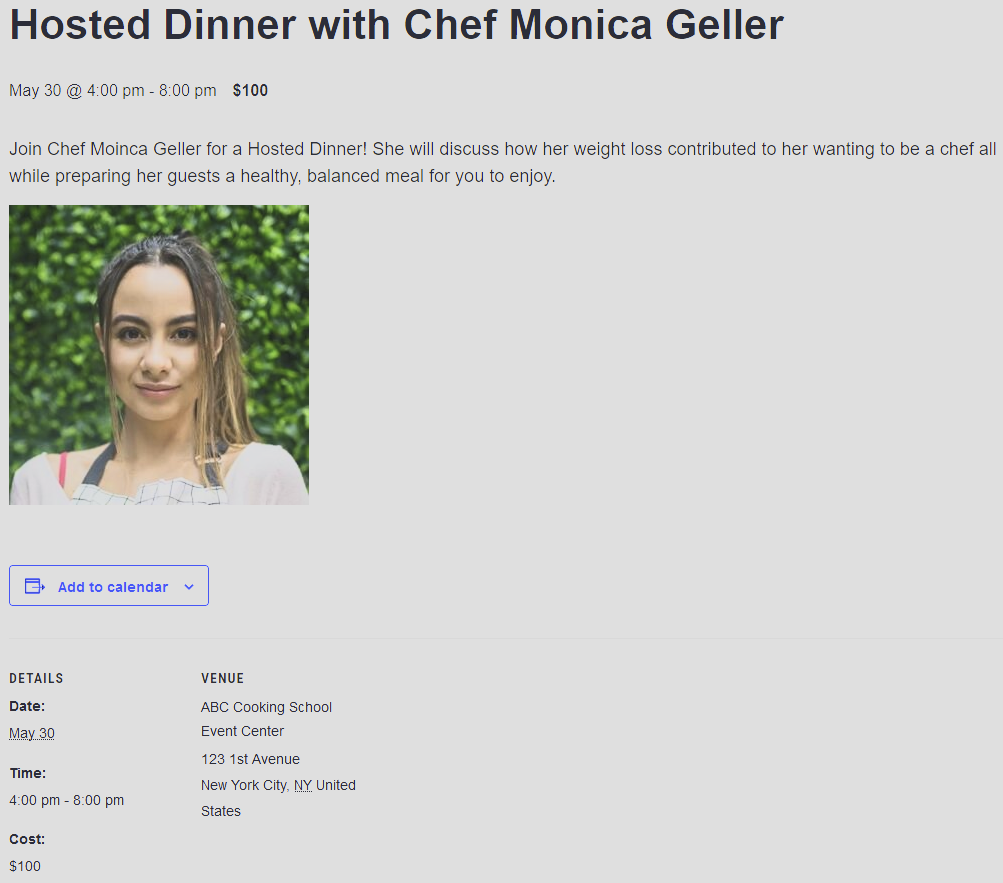
To provide our bots with the necessary time-based context, we need to sync start and end dates.
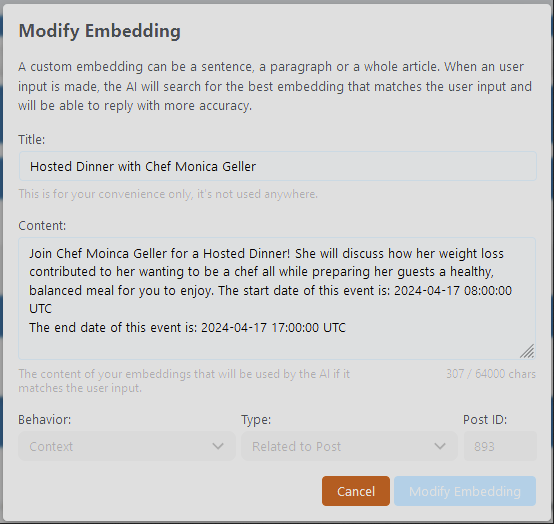
Installation
add_action('plugins_loaded', function() { global $mwai_core; if (class_exists('MeowPro_MWAI_Embeddings') && $mwai_core->get_option( 'module_embeddings', false )) { function add_date_time_to_event_embedding($content,$postId) { // Check if the post is an event if (function_exists('tribe_get_start_date') && get_post_type($postId) === 'tribe_events') { // Fetch start and end dates using the plugin's functions or WordPress get_post_meta() $timezone = date_default_timezone_get(); $start_date = tribe_get_start_date($postId, false, 'Y-m-d H:i:s'); $end_date = tribe_get_end_date($postId, false, 'Y-m-d H:i:s'); $url = get_permalink( $postId ); // Prepare the date strings $date_string = "The link to this event is here: $url.\nThe start date of this event is: $start_date $timezone"; $date_string .= "\nThe end date of this event is: $end_date $timezone"; // Append to the content $content .= "\n\n" . $date_string; } global $mwai_core; return $mwai_core->clean_sentences( $content ); } class My_Custom_Embeddings extends MeowPro_MWAI_Embeddings { function sync_vector( $vector = null, $postId = null, $envId = null ) { global $mwai_core; global $mwai; $ref = new ReflectionClass($this); if ( $postId ) { $previousVectors = parent::get_vectors_by_refId( $postId, $envId ); if ( count( $previousVectors ) > 1) { error_log( "There are more than one vector with the same refId ({$postId}). It is not handled yet." ); return; } else if ( count( $previousVectors ) === 1) { $vector = $previousVectors[0]; } else { // It's a new vector. $post = $mwai_core->get_post( $postId ); if ( !$post ) { return; } // Prepare and return the addition of a new vector based on the provided postId. $content = add_date_time_to_event_embedding($post['content'],$postId); ############# CHANGED return parent::vectors_add( [ 'type' => 'postId', 'title' => $post['title'], 'refId' => $post['postId'], 'refChecksum' => $post['checksum'], 'envId' => !empty( $envId ) ? $envId : $ref->sync_post_envId, 'content' => $content, 'behavior' => 'context' ], 'ok' ); } } // Proceed with the original function logic if $postId is not provided. if ( is_numeric( $vector ) ) { $vector = parent::get_vector( $vector ); } // If the vector does not have a refId, it is not linked to a post, and only need to be updated. if ( empty( $vector['refId'] ) ) { return parent::update_vector( $vector ); } $matchedVectors = parent::get_vectors_by_refId( $vector['refId'], $vector['envId'] ); if ( count( $matchedVectors ) > 1 ) { // Handle multiple vectors related to the same post. error_log( "There are more than one vector with the same refId ({$vector['refId']}). It is not handled yet." ); return; } $matchedVector = $matchedVectors[0]; $post = $mwai_core->get_post( $matchedVector['refId'] ); if ( !$post ) { return; } // Continue with the deletion of the matched vector and addition of the new vector. $content = add_date_time_to_event_embedding($post['content'],$postId); ############# CHANGED if ( !$ref->force_recreate && $post['checksum'] === $matchedVector['refChecksum'] && $matchedVector['status'] === 'ok') { return $matchedVector; } parent::vectors_delete( $matchedVector['envId'], [ $matchedVector['id'] ] ); // Rewrite the content if needed. if ( $ref->rewrite_content && !empty( $ref->rewrite_prompt ) ) { global $mwai; $prompt = str_replace( '{CONTENT}', $content, $ref->rewrite_prompt ); $prompt = str_replace( '{TITLE}', $post['title'], $prompt ); $prompt = str_replace( '{URL}', get_permalink( $post['postId'] ), $prompt ); $prompt = str_replace( '{EXCERPT}', $post['excerpt'], $prompt ); $prompt = str_replace( '{LANGUAGE}', $mwai_core->get_post_language( $post['postId'] ), $prompt ); $prompt = str_replace( '{ID}', $post['postId'], $prompt ); $content = $mwai->simpleTextQuery( $prompt, [ 'scope' => 'text-rewrite' ] ); } return parent::vectors_add( [ 'type' => 'postId', 'title' => $post['title'], 'refId' => $post['postId'], 'refChecksum' => $post['checksum'], 'envId' => $envId ? $envId : $matchedVector['envId'], 'content' => $content, 'behavior' => 'context' ], 'ok' ); } } global $mwai_embeddings; $mwai_embeddings = new My_Custom_Embeddings(); } },999); add_action( 'rest_api_init', function() { global $mwai_core; if (isset($mwai_core) && $mwai_core->get_option( 'module_embeddings', false )) { global $mwai_embeddings; $mwai_embeddings = new My_Custom_Embeddings(); register_rest_route( 'mwai/v1', '/vectors/sync', array( 'methods' => 'POST', 'permission_callback' => array( $mwai_core, 'can_access_settings' ), 'callback' => array( $mwai_embeddings, 'rest_vectors_sync' ), ), true ); } },999);
With start and end dates synced, the final step is to dynamically append the current date and time to the chatbot’s instructions ($query->instructions) for each user query.
// Let's add the current date and time to the bottom of our chatbot's instructions add_filter('mwai_ai_query', function ($query) { if (!($query instanceof Meow_MWAI_Query_Text) || $query->scope != 'chatbot') { return $query; } $date_string = current_time('Y-m-d H:i:s',true); $string = "# **The current date and time is $date_string.**"; if (isset($query->assistantId) && !empty($query->assistantId)) { if (!isset($query->context) || empty($query->context)) { $query->context = $string; } else { $query->context .= "\n\n".$string; } } else { $query->instructions .= "\n\n".$string; } return $query; }, 10, 1);
Success!
Your synced events now carry essential start and end date metadata, and your chatbots are fully time-aware.
Remember, this embedding module extension is your playground – customize it with WooCommerce attributes or any other metadata you desire!
Let’s enhance your business! I can help you develop custom AI engine extensions or broader AI strategies.