This code runs a free OpenAI moderation check against both the query sent to your image generator as well as the generated image itself. Anything that scores above your thresholds gets rejected.
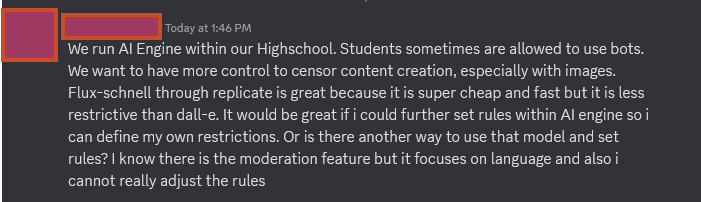
<?php // Define minimum thresholds for each category - scale of 0.0 to 1.0 $GLOBALS['safety_thresholds'] = array( 'sexual' => 0.015, 'sexual/minors' => 0.01, 'harassment' => 0.015, 'harassment/threatening' => 0.015, 'hate' => 0.015, 'hate/threatening' => 0.015, 'illicit' => 0.015, 'illicit/violent' => 0.015, 'self-harm' => 0.015, 'self-harm/intent' => 0.015, 'self-harm/instructions' => 0.015, 'violence' => 0.015, 'violence/graphic' => 0.015 ); add_filter('mwai_ai_query', function($query) { if ($query instanceof Meow_MWAI_Query_Image) { global $mwai_core; $openai = Meow_MWAI_Engines_Factory::get_openai( $mwai_core ); $result = $openai->execute('POST', '/moderations', [ 'model' => 'omni-moderation-latest', 'input' => $query->message ]); if ( !empty( $result ) && !empty( $result['results'] ) ) { $scores = $result['results'][0]['category_scores']; // Check if any category exceeds its threshold foreach ($GLOBALS['safety_thresholds'] as $category => $threshold) { if (isset($scores[$category]) && $scores[$category] > $threshold) { $score = round($scores[$category] * 100); throw new Exception(sprintf( 'Image query rejected - Content flagged for %s with confidence score of %d%%', str_replace(['/', '-'], ' ', ucfirst($category)), $score )); } } } } return $query; }, 10, 1); add_filter('mwai_ai_reply', function($reply, $query) { if ($query instanceof Meow_MWAI_Query_Image) { $images = array(); if (isset($reply->results) && is_array($reply->results)) { foreach ($reply->results as $result) { if (is_string($result) && filter_var($result, FILTER_VALIDATE_URL)) { $images[] = $result; } elseif (is_string($result) && preg_match('/https?:\/\/[^\s<>"]+/i', $result, $matches)) { $images[] = $matches[0]; } } } if (isset($reply->result)) { if (is_string($reply->result) && filter_var($reply->result, FILTER_VALIDATE_URL)) { $images[] = $reply->result; } elseif (is_string($reply->result) && preg_match('/https?:\/\/[^\s<>"]+/i', $reply->result, $matches)) { $images[] = $matches[0]; } } $images = array_unique($images); if (empty($images)) { return $reply; } $input = array(); foreach ($images as $image) { $input[] = [ 'type' => 'image_url', 'image_url' => [ 'url' => $image ] ]; } global $mwai_core; $openai = Meow_MWAI_Engines_Factory::get_openai( $mwai_core ); $result = $openai->execute('POST', '/moderations', [ 'model' => 'omni-moderation-latest', 'input' => $input ]); if ( !empty( $result ) && !empty( $result['results'] ) ) { $scores = $result['results'][0]['category_scores']; // Check if any category exceeds its threshold foreach ($GLOBALS['safety_thresholds'] as $category => $threshold) { if (isset($scores[$category]) && $scores[$category] > $threshold) { $score = round($scores[$category] * 100); throw new Exception(sprintf( 'Image rejected - Content flagged for %s with confidence score of %d%%', str_replace(['/', '-'], ' ', ucfirst($category)), $score )); } } } } return $reply; }, 10, 2);
Let’s enhance your business! I can help you develop custom AI engine extensions or broader AI strategies.
Leave a Reply