A lower temperature (closer to 0) makes the form’s responses more predictable, logical, and closer to the most likely outcomes based on its training data. Higher temperatures (closer to 1) increase the randomness, creativity, and diversity of the responses, but can also lead to less coherent or relevant answers.
Installation
Add the following JavaScript to a post or page with an AI Engine form on it:
jQuery(document).ready(function() { var input = document.querySelector('input[name="TEMPERATURE"]'); input.type = 'range'; input.min = '0'; input.max = '1'; input.step = '0.1'; input.value = '0.5'; // Set your default temperature value here var event = new Event('change', { bubbles: true }); input.dispatchEvent(event); // Create a label element var label = document.createElement('label'); label.setAttribute('for', input.id); label.textContent = input.value; jQuery(label).css("padding-left","10px"); // Create a container <div> for the slider and label var container = document.createElement('div'); container.style.display = 'flex'; container.style.alignItems = 'center'; // Align items vertically in the center // Insert the container before the input input.parentNode.insertBefore(container, input); // Move the input and label into the container container.appendChild(input); container.appendChild(label); // Update the label on input value change input.addEventListener('input', function() { label.textContent = this.value; }); });
Then add the following PHP snippet:
add_filter( 'mwai_ai_query', function ( $query ) { if ($query->scope != 'form' || !isset($query->extraParams) || !isset($query->extraParams['fields']) || !isset($query->extraParams['fields']['TEMPERATURE'])) { return $query; } $temperature = $query->extraParams['fields']['TEMPERATURE']; $prefix = '['.$temperature.']'; if (strncmp($query->message, $prefix, strlen($prefix)) === 0) { $query->message = substr($query->message, strlen($prefix)); } $query->temperature = max(0.0, min(1.0, (float)$temperature)); return $query; }, 1, 1);
Optionally, edit the JavaScript and change input.value = '0.5'; // Set your default temperature value here
Create an input field with the name TEMPERATURE with the field type of Input.
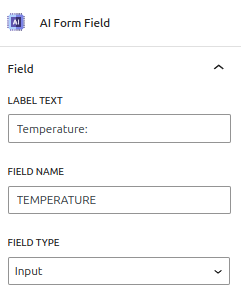
Then add [{TEMPERATURE}] to the beginning of the prompt on the submit element. It will be removed before being sent to your connected AI model.
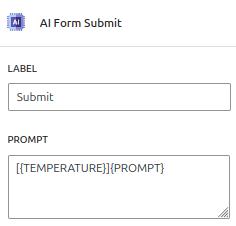
Let’s enhance your business! I can help you develop custom AI engine extensions or broader AI strategies.
Contact me.